Quick Tips: Pointer Optimizations in Go
Overview
This article explores important performance considerations when working with pointers in Go. We'll cover key topics like returning pointers to local variables, choosing between pointer and value receivers for methods, and how to properly measure and optimize pointer-related performance using Go's built-in tools. Whether you're new to Go or an experienced developer, these tips will help you write more efficient and maintainable code.
Target Audience
This article is designed for:
- Intermediate Go developers looking to deepen their understanding of pointer performance
- Developers transitioning to Go from other languages who need to understand Go's unique approach to pointers
- Performance-focused engineers who want to optimize their Go applications
- Team leads and code reviewers who need to evaluate pointer-related design decisions
- Anyone interested in Go's memory management and performance characteristics
Prior experience with Go basics and familiarity with fundamental programming concepts is assumed. While we touch on advanced topics like escape analysis and profiling, these concepts are explained in an accessible way.
I was recently updating some course material and noticed several small but useful pointer tricks I've learned over the years in Go. While these optimizations aren't complicated, they're often not well known. It seemed like a good time to bring these tips together into a blog post.
Returning local variables from functions as pointers
When developers transition to Go from other programming languages, they often bring along practices and assumptions about memory management that might not align with Go's approach. One common scenario that frequently surprises newcomers is how Go handles returning pointers to local variables. Let's explore this concept through a common pattern that, while functional in Go, can be misleading and potentially confusing for developers and code reviewers.
Consider this function:
func meaningOfLife () *int {
x := 42
return &x // Surprisingly OK in Go! Compiler handles this
}
In many programming languages, returning a pointer to a local variable is considered bad practice because the local variable's memory is typically deallocated once the function exits. Accessing this memory afterward can lead to undefined behavior, such as accessing garbage values or causing a program crash.
However, Go takes a different approach. The compiler performs escape analysis to determine the lifetime of variables. If it detects that a local variable's address is being returned, it allocates the variable on the heap instead of the stack. This ensures that the variable remains valid even after the function exits.
While meaningOfLife()
might appear harmless due to Go's automatic escape analysis, it can be misleading about memory management. The code suggests the variable x
is stack-allocated, but it actually escapes to the heap. This implicit behavior can mask potential performance implications and make it harder for developers to reason about memory patterns, especially in larger codebases where understanding garbage collection patterns becomes crucial. The garbage collector will need to track and manage this escaped variable, even though the code's structure implies stack allocation.
A more explicit and clearer approach would be:
// Returning pointer to a heap-allocated variable
func meaningOfLife() *int {
x := new(int)
*x = 42
return x
}
In this improved version, new(int)
explicitly allocates memory on the heap, making it clear that the returned pointer will remain valid after the function exits. This approach improves code readability and maintainability.
Additional considerations for new Go developers:
- Performance Impact: While the difference might be negligible for simple cases, in hot paths or high-performance code, explicit heap allocations make it easier to profile and optimize memory usage.
- Code Review: Explicit heap allocations make code reviews more effective as the intended memory behavior is clear.
- Debug Tooling: Tools like
go build -gcflags='-m'
can help identify when variables escape to the heap, which is valuable for understanding memory behavior. - Stack vs Heap: Remember that stack allocations are generally faster and put less pressure on the garbage collector. When possible, prefer stack allocations for short-lived variables that don't need to outlive the function.
- Interface Values: Be particularly careful with interface values, as they can cause unexpected heap allocations when storing concrete types.
Pointer vs Value Receivers
One of Go's powerful features is its method receiver system, which allows developers to define methods on types. However, the choice between pointer receivers and value receivers can have significant implications for both program behavior and performance. Let's explore these implications and understand how to make informed decisions about receiver types.
Consider this example of a large struct with different method implementations:
type LargeStruct struct {
data [1024]int
metadata string
timestamp time.Time
status uint32
// ... potentially many more fields
}
// Good: Avoids copying large struct
func (l *LargeStruct) process() {
// Process data without creating a copy
}
// Bad: Copies entire struct
func (l LargeStruct) processCopy() {
// Process data, but entire struct is copied
}
Impact on Memory and Performance
When using a value receiver, Go makes a complete copy of the struct for the method call. For small structs (a few basic fields), this copying overhead is negligible. However, for larger structs, especially those containing arrays or slices, this copying can become a significant performance bottleneck:
- Memory Overhead: Each method call with a value receiver creates a new copy of the entire struct, temporarily doubling the memory usage for that struct.
- CPU Overhead: The time spent copying large data structures can accumulate, especially in tight loops or frequently called methods.
- Cache Impact: Large struct copies can lead to cache misses, affecting not just the method's performance but potentially the entire program's performance.
Guidelines for Choosing Receivers
While it might be tempting to always use pointer receivers for large structs, the decision should be based on several factors:
- Struct Size: For small structs (usually less than 64 bytes), the copy overhead is minimal, and value receivers might be preferable for better cache locality.
- Method Behavior:
- Use pointer receivers when the method needs to modify the receiver
- Use pointer receivers for large structs to avoid copying
- Use pointer receivers for consistency if any method requires a pointer receiver
- Concurrency Considerations: Value receivers provide natural protection against race conditions since each goroutine works with its own copy. Note: This could also be a potentical bug if you need to ensure you are always working with the latest copy of the value. Make sure you understand the needs of your program before accepting this as a "good" thing.
The Importance of Measurement in Go Performance Optimization
While the guidelines above provide a good starting point, it's crucial to emphasize that performance optimization should always be driven by data, not assumptions. Go provides excellent tools for measuring and profiling your application's performance:
Benchmarking Your Code
func BenchmarkLargeStructMethods(b *testing.B) {
ls := LargeStruct{}
b.Run("Pointer Receiver", func(b *testing.B) {
for i := 0; i < b.N; i++ {
ls.process()
}
})
b.Run("Value Receiver", func(b *testing.B) {
for i := 0; i < b.N; i++ {
ls.processCopy()
}
})
}
Using Go's Profiling Tools
- CPU Profiling: Use
runtime/pprof
ornet/http/pprof
to identify CPU-intensive operations - Memory Profiling: Track allocations and identify memory patterns
- Escape Analysis: Use
go build -gcflags='-m'
to understand when variables escape to the heap
Best Practices for Performance Optimization
- Measure First: Always establish a baseline performance measurement before making optimizations
- Profile in Production-Like Conditions: Test with realistic data sizes and loads
- Consider the Full Context:
- Is this code in a critical path?
- How frequently is it called?
- What are the actual memory and CPU constraints?
- Document Performance Decisions: When making performance-based choices, document the measurements that led to those decisions
Avoiding Premature Optimization
Remember the famous quote: "Premature optimization is the root of all evil." Before investing time in optimizing receiver types or any other performance-related changes:
- Verify the Problem: Use profiling tools to confirm that the method receiver choice is actually impacting performance
- Measure the Impact: Quantify the performance difference between implementations
- Consider Maintenance: Sometimes slightly lower performance is acceptable if it leads to clearer, more maintainable code
- Test Real-World Scenarios: Micro-benchmarks might not reflect real-world performance characteristics
By following these principles and using Go's built-in tools for performance analysis, you can make informed decisions about method receivers and other performance optimizations, ensuring that your code is both efficient and maintainable.
More Articles
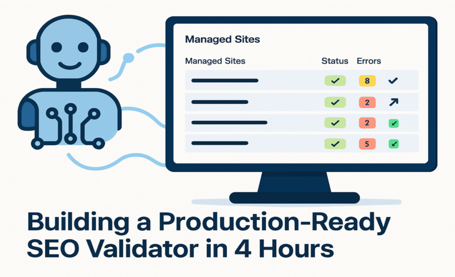
Building a Production-Ready SEO Validator in 4 Hours
Overview
As a senior developer with 20+ years in the trenches, I built a fully functional, production-ready SEO validation system in under 4 hours using AI assistance. Ten years ago, this would have taken me weeks. But here's the key insight: without being a senior level developer, AI would have never gotten this to work. This is the story of how AI amplifies expertise rather than replacing it, complete with real metrics, mistakes made, and lessons learned.
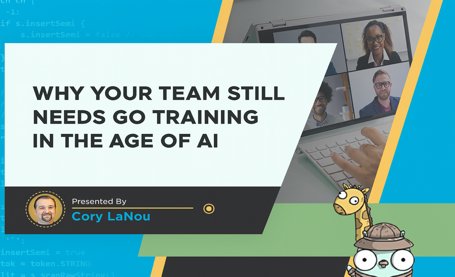
Why Your Team Still Needs Go Training in the Age of AI
Overview
AI hasn't killed software training. In fact, after seeing training demand slow down initially when ChatGPT and Copilot took off, we're now busier than ever. Why? Because teams are discovering what I've been saying all along - AI is only as good as the code it learned from, and most Go code out there isn't idiomatic.
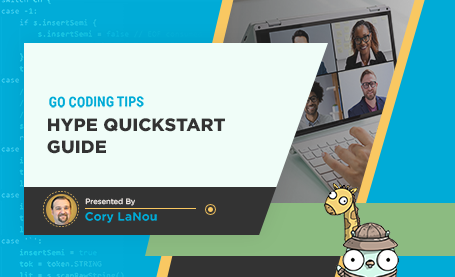
Hype Quick Start Guide
Overview
This article covers the basics of quickly writing a technical article using Hype.